Loading... # Vue(一) ## Vue基础 ### 简介 * JavaScript框架 * 简化Dom操作 * 响应式数据驱动 ### 第一个Vue程序 ~~~html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Vue</title> </head> <body> <div id="app"> {{ message }} </div> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script> var app = new Vue({ el:"#app", data: { message: "Hello Vue!" } }) </script> </body> </html> ~~~ 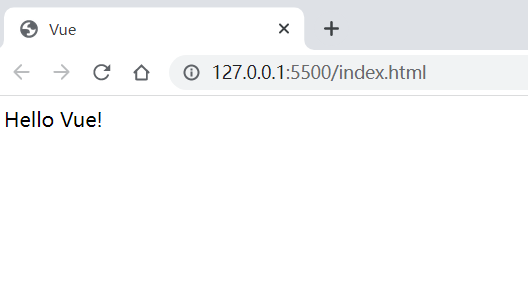 步骤 * 导入开发版本的Vue.js * 创建Vue实例对象,设置el属性和data属性 * 使用简介的模板语法把数据渲染到页面上 ### el:挂载点 Vue实例作用范围:管理el选项命中的元素及其内部的后代元素 ~~~ id: el:"#app" class: el:".app" ~~~ **建议使用id选择器** vue不能挂载到html、body标签上 ### data数据对象 ~~~html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Vue</title> </head> <body> <div id="app"> <h1>{{ info.message }} by {{ info.author }}</h1> <h2>from {{ loc[0] }}</h2> </div> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script> var app = new Vue({ el:"#app", data: { info:{ message: "Hello Vue!", author: "Xherlock" }, loc:["成都","北京","上海","郑州","伦敦","威尼斯"] } }) </script> </body> </html> ~~~ * Vue中用到的数据定义在data中 * data中可以写复杂类型的数据 * 渲染复杂类型数据时遵守js语法即可 ## 本地应用 ### v-text 设置标签的文本值(textContent),差值表达式:{{}} eg:\<h1 v-text="message">\</h1>,想要加入其他字符在双引号中+'xxx'单引号包含要加的内容 ~~~html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Vue</title> </head> <body> <div id="app"> <h1 v-text="message+'?'"></h1> <h2 v-text="author+'!'"></h2> </div> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script> var app = new Vue({ el:"#app", data: { message: "Hello Vue!", author: "Xherlock" } }) </script> </body> </html> ~~~ 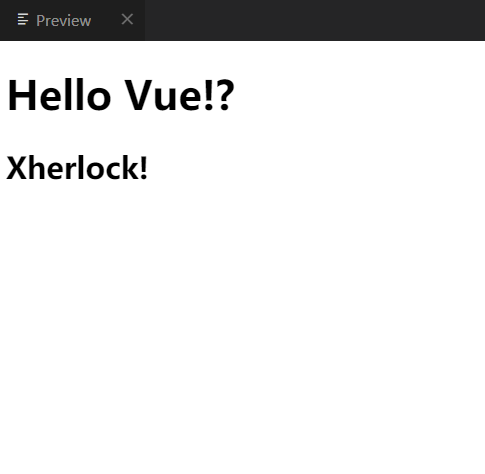 ### v-html 设置标签的innerHTML eg:\<p v-html="content">\</p> ~~~html <body> <div id="app"> <p v-html="content"></p> </div> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script> var app = new Vue({ el:"#app", data: { content: "<a href='http://xherlock.top'>Hello Vue!</a>", author: "Xherlock" } }) </script> </body> ~~~ 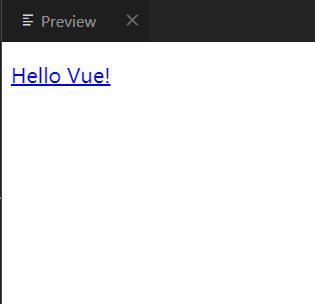 ### v-on 为元素绑定事件 eg: ~~~html <input type="button" value="事件绑定" v-on:click="方法"> <input type="button" value="事件绑定" v-on:monseenter="方法"> <input type="button" value="事件绑定" v-on:dblclick="方法"> ~~~ ~~~js methods:{ // 不要少了s doIt:function(){ // 逻辑 } } ~~~ ~~~ <body> <div id="app"> <input type="button" value="v-on指令" v-on:click="show()"> <input type="button" value="v-on简写" @click="show()"> <input type="button" value="双击事件" @dblclick="show()"> </div> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script> var app = new Vue({ el:"#app", methods: { show:function(){ alert("Xherlock"); } } }) </script> </body> ~~~  * 事件名不需要写on * 指令可以简写为@ * 绑定的方法定义在methods属性中 * 方法内部通过this关键字可以访问定义在data中的数据 ### 计数器 ~~~html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Vue</title> </head> <body> <div id="app"> <div class="calc"> <button @click="sub"> - </button> <span>{{num}}</span> <button @click="add"> + </button> </div> </div> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script> var app = new Vue({ el:"#app", data: { num: 0 }, methods: { sub:function(){ if(this.num>0){ this.num--; }else{ alert("别减了,到底了!") } }, add:function(){ if(this.num<10){ this.num++; }else{ alert("别加了,到顶了!") } } } }) </script> </body> </html> ~~~ 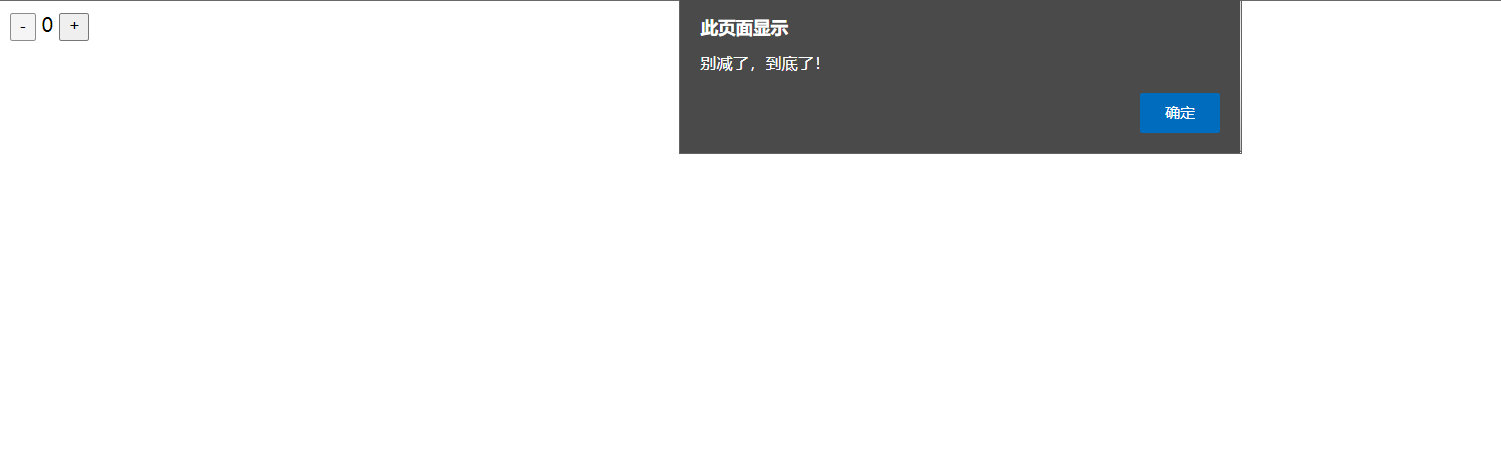 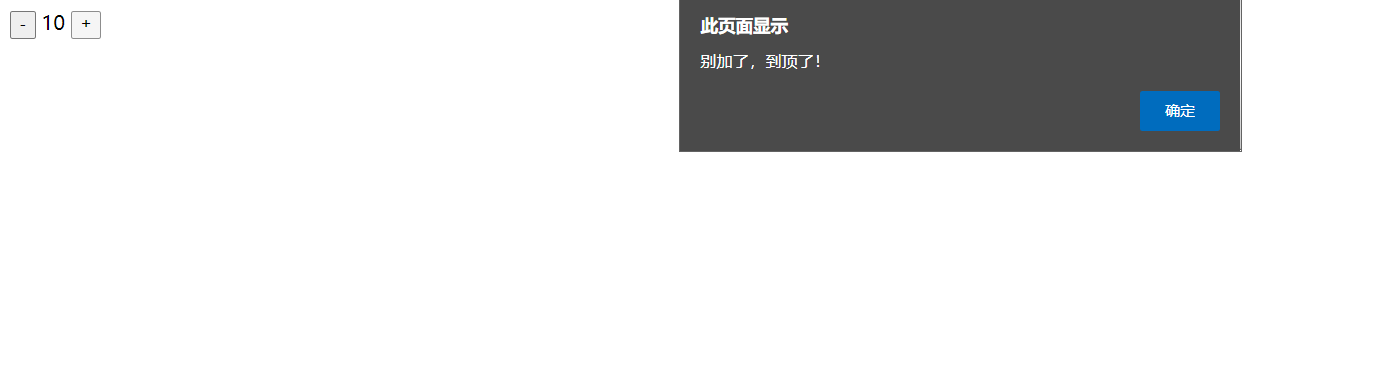 ### v-show 根据表达值的真假,切换元素的显示与隐藏 eg:\<img v-show="false" src="./xxx.png"\>,不显示图片 ~~~html <body> <div id="app"> <input type="button" value="切换显示状态" @click="change"> <img src="./1.jpg" alt="captain" v-show="isShow"style=""> </div> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script> var app = new Vue({ el:"#app", data: { isShow: false }, methods: { change:function(){ this.isShow = !this.isShow; } } }) </script> </body> ~~~ 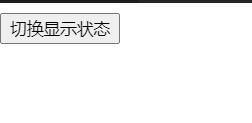 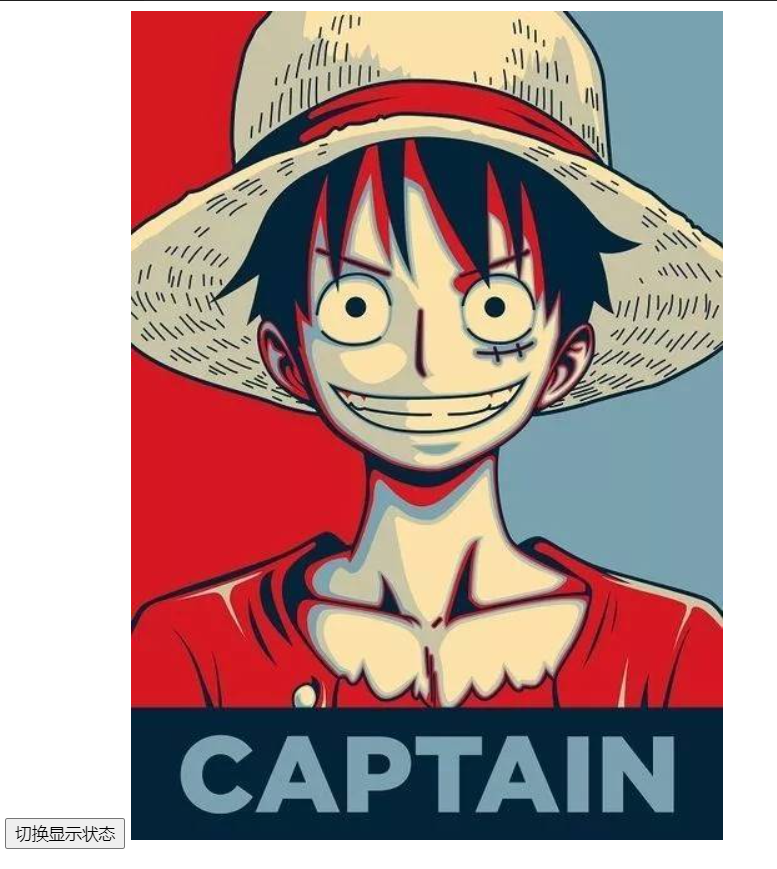 * 原理是修改元素的display,实现显示和隐藏 * 指令后面的内容,最终都会解析成布尔值 * 值为true元素显示,false元素隐藏 * 数据改变之后,对应元素的显示状态会同步更新 最后修改:2022 年 03 月 29 日 © 允许规范转载 打赏 赞赏作者 支付宝微信 赞 0 如果觉得我的文章对你有用,请随意赞赏